Documentation as a Code
Documentation is an integral part of any project. No matter how agile you are, you must explain what you are doing.
Markdown
The idea of using special characters to format text is not new. Computers use non-printing bytes to control how text is presented on the terminal. Everyone knows that the 0x0A
character is called a "Line Feed" and is used as the end of a text line. Sometimes, a new line ends with the 0x0D
(carriage return) character. These two bytes were initially used together (transition to a new line and return the cursor to the first column). Other ASCII characters have been used to control the flow of text: 0x09
(tab), 0x1E
(record separator), and 0x03
(end of text). What about 0x07
? Doesn't that ring a bell?
In the late 1970s, the creators of the once-popular word processor WordPerfect extended this idea. The application used special codes like “italics on” and “italics off” to format text. This approach was very flexible but xhighly inconvenient for users.
Microsoft Word changed the game circa 1983. It provided users with a most desirable feature: simplicity. Type text, press ENTER only at the end of a paragraph, and the program will word-wrap it automatically. You can format the text (bold, italics), and you can change the alignment of paragraphs—left, right, centered, and justified. That's it! Over the past 40 years, the simple program has evolved into the Microsoft Office suite, but the simplicity has been lost.
In 2004, in collaboration with Aaron Schwartz, John Gruber proposed a simple concept for a simple, human-readable syntax for writing text that could be easily converted to HTML. They called it Markdown.
The original syntax included just a few rules. The idea is that you don't need a heavy office application to write text containing headings, highlights (bold and italics), or formatted code (variableExampleName
). Simple markdown also supports:
- Blockquotes,
- Lists (both numbered and bullets),
- HTTP links,
- Embedded Images (either local or from the web),
- code blocks.
Today, most of the programming text editors and IDEs support markdown syntax. You can find many plugins to preview the formatted version or edit it in WYSIWYG mode.
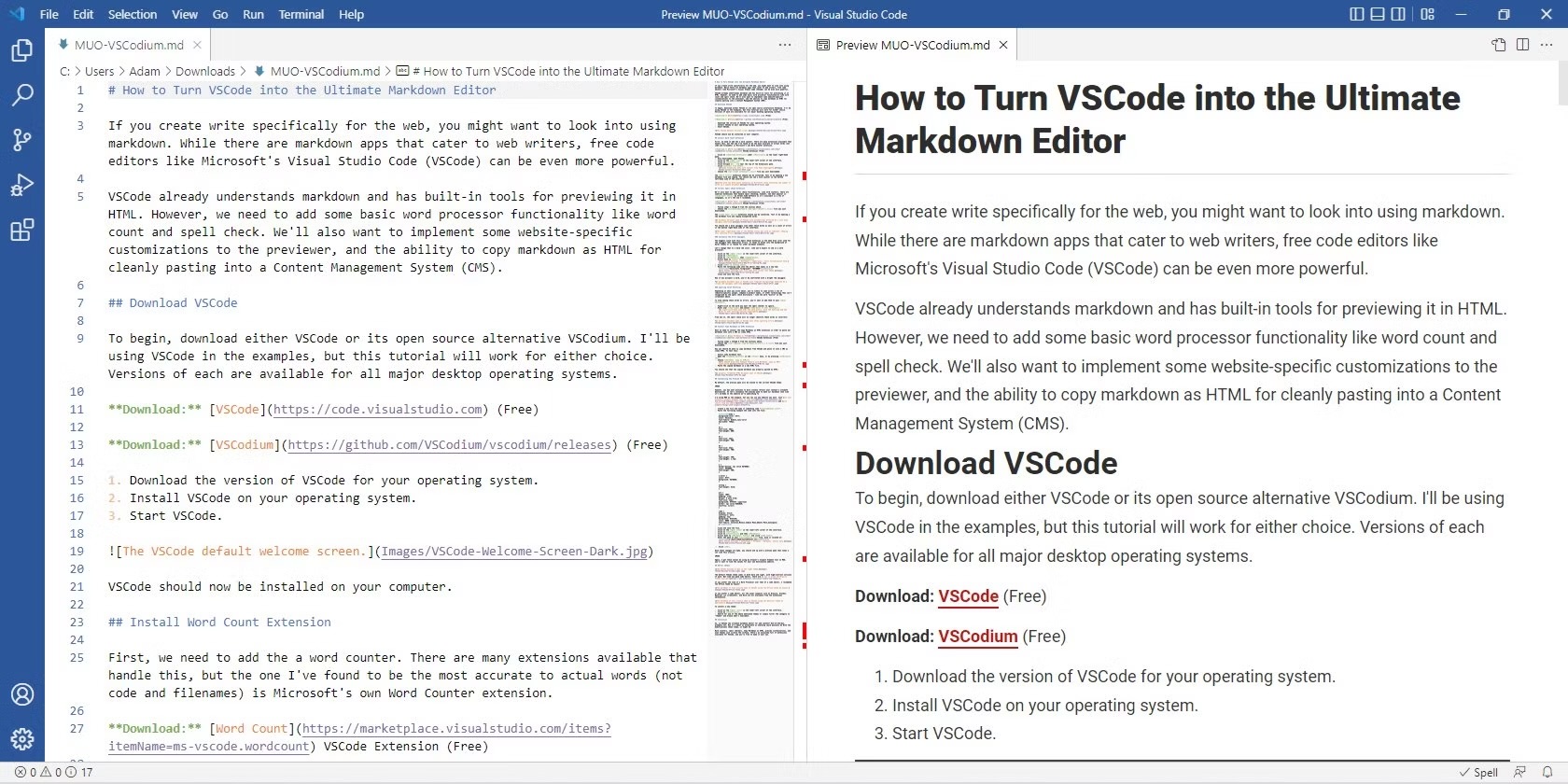
Using Markdown in VS Code
Source code repositories such as Github, Gitlab, Bitbucket, etc. automatically format markdown texts. To learn more about markdown basic syntax, read this page on Markdown Guide website.
Extended Syntax
Once developers got a taste for the convenience of Markdown, they wanted to do all their writing in this format. Markdown's extended syntax has the ambitious goal of let you to abandon bloated word processors and do all your writing in the text editor of your choice.
To say goodbye to Microsoft Word, we needed the ability to add tables. Markdown's advanced syntax supports this functionality. Drawing tables manually in text format is no fun, but applications such as Typora make this task much easier.
Extended syntax also supports fenced code blocks and code syntax indicators. Now, you can specify the code language, and the page viewer will highlight it. For example, this is a NodeJS code:
const main = async () => {
try {
const data = await fsPromises.readFile(filePath);
const obj = JSON.parse(data);
console.log(obj)
} catch (err){
console.log(err);
}
}
The extended markdown is supported by this Wiki:
GitHub | GitLab | Typora | |
---|---|---|---|
Basic Syntax | ✔ | ✔ | ✔ |
Tables | ✔ | ✔ | ✔ |
Syntax highlighting | ✔ | ✔ | ✔ |
Task List | ✔ | ✔ | ✔ |
Strikethrough ( |
✔ | ✔ | ✔ |
Highlight | ✔ | ✔ | – |
Emoji Shortcodes (⛺️) | ✔ | ✔ | – |
Subscript/Superscript | ✔ | ✔ | – |
With the rapid spread of technology, we can expect to see more extensions to this format. As long as these features can be used in plain text, the complexity will be kept at a manageable level.
To learn more about markdown extended syntax, read this page on Markdown Guide website.
Diagrams
Once we have decoupled ourselves from Microsoft Word, the next step is to replace PowerPoint and Visio. Technical documentation needs lots of pictures to visualize complex aspects of architecture and design. To claim a solution called “documentation as a code,” we need diagrams. Many tools can provide this capability.
GraphViz
Graphviz is an open-source graph design software. It has been a long-standing tool in the field of code-sourced graph visualization. Here is an example of a simple GraphViz diagram:
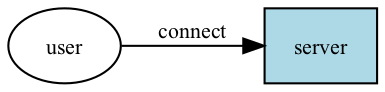
GraphViz Diagram
The picture above represents the following code:
digraph Demonstrate {
rankdir=LR;
node [ style=filled; fillcolor="white"; ]
user [shape=oval];
server [shape=box, fillcolor="lightblue"];
user -> server [label="connect"];
}
You can edit GraphViz diagrams online at GitHub. You can also download the GraphViz compiler and convert diagram code files (extension .dot
) to PNG or PDF files. To learn more about GraphViz syntax and tools, visit the graphviz.org website.
PlantUML
PlantUML is another open-source tool that aims to provide code-based specifications for UML diagrams. PlantUML provides a plain text syntax and tools for converting code to images. You can draw almost any architectural diagram you need when designing a system. Here are just some of the types of diagrams you can create:
- Sequence diagram
- Usecase diagram
- Class diagram
- Activity diagram
- Component diagram
- State diagram
- Object diagram
- Deployment diagram
- Timing diagram
Here is an example of the PlantUML sequence diagram:
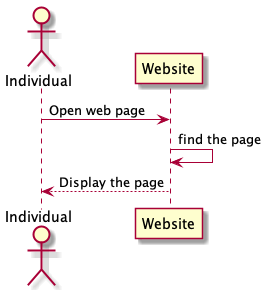
PlantUML Diagram
The picture above represents the following code:
@startuml
actor "Individual" as User
participant "Website" as Site
User -> Site: Open web page
Site -> Site: find the page
Site --> User: Display the page
@enduml
You can edit PlantUML diagrams online at the planttext.com. You can also download the PlantUML compiler and convert diagram code files (extension .puml
) to PNG or PDF files.
To learn more about PlantUML syntax and tools, visit the plantuml.com website.
Mermaid.JS
The later addition to code-driven diagramming tools is Mermaid. It is a Javascript-based diagramming and charting tool that renders Markdown-inspired text definitions to create and modify diagrams dynamically.
Many platforms, including Typora markdown editor and GitLab, support embedded Mermaid diagrams. That means you do not have to convert the diagram code to images. Instead, type your diagram code directly into your text. Here is an example of Mermaid embedded diagram:
flowchart LR A[Hard] -->|Text| B(Round) B --> C{Decision} C -->|One| D[Result 1] C -->|Two| E[Result 2]
The picture above represents the following code:
flowchart LR
A[Hard] -->|Text| B(Round)
B --> C{Decision}
C -->|One| D[Result 1]
C -->|Two| E[Result 2]
Note, the Typora supports Mermaid 8.8.3. It may not support the latest features added to Mermaid. To learn more about Mermaid syntax and tools, visit the mermaid.js.org website.
Exalidraw
What could be the final nail in the coffin of proprietary diagramming tools is Excalidraw. This is an open-source virtual whiteboard tool developed by one of the creators of React Native. The online editor allows users to create hand-drawn-like diagrams and sketches easily. The great thing about Excalidraw is that it also saves diagrams in text format.
Here is an example that shows what kind of diagrams you can create using this tool:
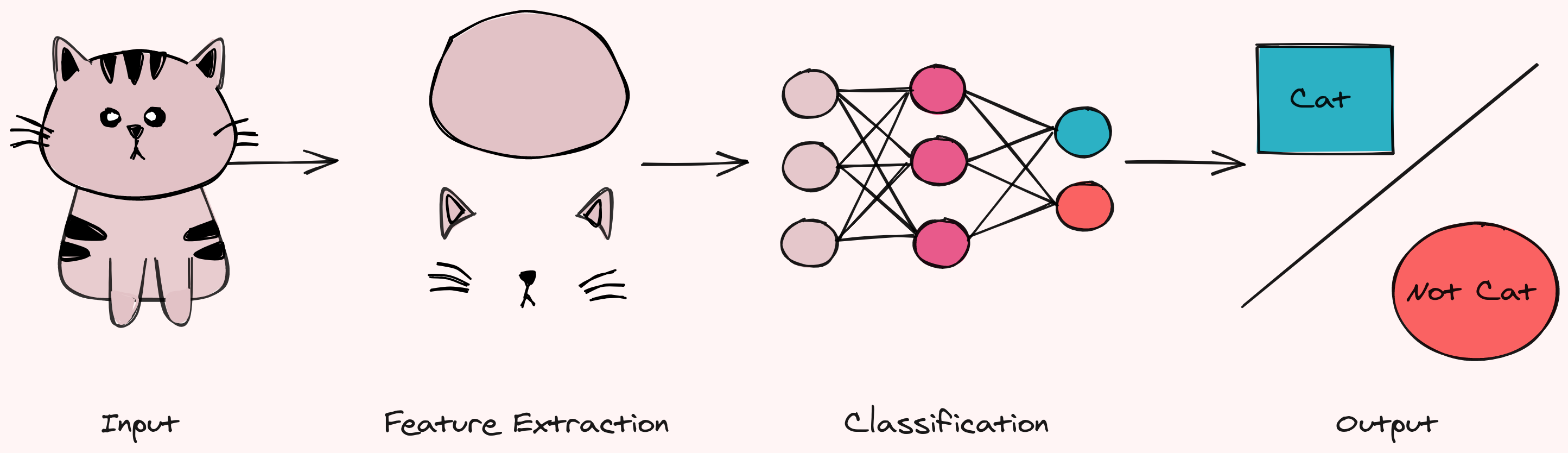
Excalidraw
The tool is free and open source. If you value your time, you have the option to use the commercial version of the product.
You can try it on Excalidraw.com website.
Conclusion
Documentation as code (DaaC) is applicable not only for creating and editing documentation. You can use these tools to create PDF documents, wikis, and even websites (like this one). There is also MARP, a presentation creation framework that converts Markdown documents into presentations. To learn more about using this tool, visit MARP website.
Using a code-driven approach to develop content offers a few significant advantages:
- You can use a text editor to search/replace, duplicate, and annotate diagram code.
- You can put diagram code into Git and manage it as any other code. Use diff to see what exactly was changed in both text and diagrams.
However, the most powerful feature of this approach is that you can write code that simplifies and automates documentation by dynamically generating markdown texts and diagrams. Recent improvements in generative AI tools can also help create content in that format. It remains editable for manual adjustments.
There is no limit to imagination.